In the image above is a famous quote from Gary Provost’s “100 Ways to Improve Your Writing”. One of them is to avoid stacking sentences of the same length one after another, since that makes for boring reading. Coloring the text based on sentence length is a useful visual tool to help an author or an editor identify such potentially boring passages and revise them. I found several web-based tools that do this (here’s an example) but I don’t much like the mechanics of pasting my text into a browser, possibly many times, as I revise. There might also be some commercial products that offer this service, but I didn’t research that.
Instead, I made my own sentence length colorizer for Microsoft Word, and if you’d like, you can use it too!
This is what it looks like on that same example:
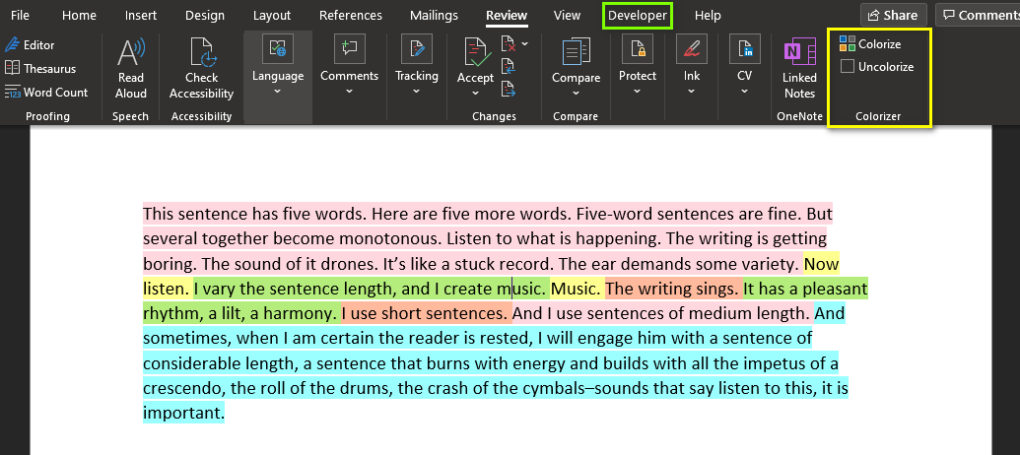
It works like this: you make a selection and press the Colorize button (in the top right corner of the screenshot) that runs a macro I wrote and colors your text based on sentence length. When you’re done surveying your handiwork, you press the Uncolorize button to make the text normal again.
Setup
- Download this zip. If you’d rather not, you can copy-paste the code (it’s listed further down) directly into your Visual Basic editor in Word (I’ll tell you how in step 7). For now, I proceed assuming you downloaded the file.
- It contains a file named SentenceLengthColorizer.bas. That’s the macro. Unpack it to a permanent location. You can inspect the file in a text editor if you wish to see (or fiddle with) the code before you plug it into Word.
- Fire up your Microsoft Word. If you see a Developer ribbon tab (like in the screenshot above), proceed to step 5.
- If you don’t, right-click an empty space on the ribbon (or go to File -> Options) and select Customize Ribbon. Locate the Developer tab in the list of main tabs on the right side, and put a check-mark in it.
- Click the Visual Basic button in the Developer tab. This will launch the Visual Basic editor window. On the left side of this window, you’ll see something like a file system tree, with your document listed under “Project” and another node titled “Normal”.
- Right click the “Normal” node, select Import File… from the context menu and point to the unpacked .bas file from step 1.
- If instead of downloading this file you opted for copy-pasting the code, choose Insert > Module instead of Import File… from the context menu. This will open a code editing window where you can paste the code copied from below.
- At this point, you can already run the macro by clicking the Macros button in the Developer tab. It will list the Colorize and Uncolorize commands that you can apply to selected text.
- To have the handy buttons, however, you have to add them in yourself. Bring up the Customize Ribbon window once again and either create a New Tab for this, or a New Group in an existing tab. I added a new group to the Review tab. Name the tab/group what you want using the Rename button. I named mine “Colorizer”.
- Switch from “Popular Commands” to “Macros” on the left side, where commands are listed. You should see the two macros, Colorize and Uncolorize, in the list. Their names will be prefixed with the name of the macro file (SentenceLengthColorizer) and possibly your document template (Normal) so their names might not be fully visible.
- With the new group you created in step 9 selected in the list on the right, select the Colorize command in the list on the left and click the Add >> button. Do the same with the Uncolorize command. Then use the Rename button to give them names and icons of your choice. Voila! You can now use the sentence length colorizer from your ribbon.
Customization
Things one might want to customize are the colors and the sentence length stops. Sadly, the only way to change these settings is by directly modifying the code of the macro. Here it is:
Option Base 1
Sub Colorize()
' The number of different sentence-length-based categories/colors
Const catCount As Long = 7
' The list of word counts defining the sentence-length categories
Dim wordCounts(catCount - 1) As Long
' Word counts assignment
wordCounts(1) = 3
wordCounts(2) = 5
wordCounts(3) = 10
wordCounts(4) = 20
wordCounts(5) = 30
wordCounts(6) = 50
' The list of colors matching the word counts
' Note that it has one element more than the list of word counts
Dim sntcColors(catCount) As Long
' Color assignment
' The last element is the color you want to see on sentences longer
' than the largest count in word-count list
sntcColors(1) = RGB(255, 255, 143)
sntcColors(2) = RGB(248, 142, 134)
sntcColors(3) = RGB(255, 216, 223)
sntcColors(4) = RGB(180, 237, 123)
sntcColors(5) = RGB(255, 211, 144)
sntcColors(6) = RGB(237, 216, 255)
sntcColors(7) = RGB(156, 255, 255)
Dim sntcColorInd As Long
For Each sntc In Selection.Sentences
With sntc
sntcColorInd = catCount
For ind = 1 To catCount - 1
If .Words.Count <= wordCounts(ind) Then
sntcColorInd = ind
Exit For
End If
Next
With .Font.Shading
.Texture = wdTextureNone
.ForegroundPatternColor = wdColorAutomatic
.BackgroundPatternColor = sntcColors(sntcColorInd)
End With
End With
Next
End Sub
Sub Uncolorize()
Selection.Font.Shading.BackgroundPatternColorIndex = wdAuto
End Sub
Code language: VB.NET (vbnet)
I know this might seem intimidating for people without coding experience, but bear with me.
On line 9, I create a list (wordCounts) of word counts for coloring sentences. They are specified on lines 12–17. The matching colors (sntcColors) are specified in a similar fashion on lines 21–32 via their RGB values. Sentences with 3 words or less (wordCounts(1)) will have one color (sntcColors(1)), those with more than 3 but less than 5 words (wordCounts(2)), another color (sntcColors(2)), and so on. The last color in (sntcColors(7)) will be applied to all sentences with more than 50 words.
The number of categories (how many different colors you wish to see applied on your text) is defined on line 6 as catCount. For the macro to work properly, the sntcColors list must have exactly that many elements, and the wordCounts list, one element fewer.
Say you wanted to add a new color for sentences between 20 and 25 words. You’d modify the code like this:
' The number of different sentence-length-based categories/colors
Const catCount As Long = 8
' The list of word counts defining the sentence-length categories
Dim wordCounts(catCount - 1) As Long
' Word counts assignment
wordCounts(1) = 3
wordCounts(2) = 5
wordCounts(3) = 10
wordCounts(4) = 25
wordCounts(5) = 20
wordCounts(6) = 30
wordCounts(7) = 50
' The list of colors matching the word counts
' Note that it has one element more than the list of word counts
Dim sntcColors(catCount) As Long
' Color assignment
' The last element is the color you want to see on sentences longer
' than the largest count in word-count list
sntcColors(1) = RGB(255, 255, 143)
sntcColors(2) = RGB(248, 142, 134)
sntcColors(3) = RGB(255, 216, 223)
sntcColors(4) = RGB(255, 255, 0)
sntcColors(5) = RGB(180, 237, 123)
sntcColors(6) = RGB(255, 211, 144)
sntcColors(7) = RGB(237, 216, 255)
sntcColors(8) = RGB(156, 255, 255)
Code language: VB.NET (vbnet)
The rest of the code remains the same. Not that bad, eh? Just be sure that there’s as many colors as there are categories, and one less word count.
Say you wanted to decrease the number of possible colors instead, by removing the stop at 30 words. You’d then modify the code like this:
' The number of different sentence-length-based categories/colors
Const catCount As Long = 6
' The list of word counts defining the sentence-length categories
Dim wordCounts(catCount - 1) As Long
' Word counts assignment
wordCounts(1) = 3
wordCounts(2) = 5
wordCounts(3) = 10
wordCounts(4) = 20
wordCounts(5) = 50
' The list of colors matching the word counts
' Note that it has one element more than the list of word counts
Dim sntcColors(catCount) As Long
' Color assignment
' The last element is the color you want to see on sentences longer
' than the largest count in word-count list
sntcColors(1) = RGB(255, 255, 143)
sntcColors(2) = RGB(248, 142, 134)
sntcColors(3) = RGB(255, 216, 223)
sntcColors(4) = RGB(180, 237, 123)
sntcColors(5) = RGB(255, 211, 144)
sntcColors(6) = RGB(156, 255, 255)
Code language: VB.NET (vbnet)
If you’re happy with the number of the colors, but want to change the colors themselves, just paste the desired RGB values on lines 24–30.
Known and potential issues
This is by no means foolproof or well-tested. I’ll list some issues that I came across while using it, and those I can imagine happening but haven’t encountered yet.
- Colorizing doesn’t happen real-time, as you type. It applies a static effect on the selected text.
- Colorizing/uncolorizing won’t affect text highlighted using the built-in highlight tool.
- Word has some strange ideas about word counts. Sometimes the quote mark at the beginning of the sentence (for example, in dialog), or a blank line under a title will count as an additional word. This may result in two sentences with the same number of words being colored differently. There’s nothing I can do about it.
- If you Select All the text in a lengthy document, the coloring will take a while. It takes my monster machine 3-4 seconds (enough to wonder if the command worked) to color a 25000 words (50 pages) document whole.
I hope someone will find this useful! If you run into trouble (or have some other feedback), post a comment here. I’ll be delighted to hear from you and I’ll do my best to help.